Permalink
The software is written using C language and communicates with the Serial Port using Win32 API. In Windows,Serial ports are named as COM1, COM2,COM3. COM1 and COM2 usually refer to the hardware serial ports present in the PC while COM numbers in double digits like COM32, COM54,COM24. Etc are given to USB to Serial Converters or PCI. The following code example demonstrates the use of the SerialPort class to allow two users to chat from two separate computers connected by a null modem cable. In this example, the users are prompted for the port settings and a username before chatting. Both computers must be executing the program to achieve full functionality of this example. A C Class for Controlling a Serial Port in Windows. Whilst serial ports are old technology now, and has mostly been superceded by USB, it is still an easy way to communicate with embedded hardware; it is great for small projects. This page presents a simple C class for communicating with hardware via a serial port.
Join GitHub today
GitHub is home to over 40 million developers working together to host and review code, manage projects, and build software together.
Sign up1 contributor
/**/ |
/* Serial Port Programming in C (Serial Port Read) */ |
/* Non Cannonical mode */ |
/*----------------------------------------------------------------------------------------------------*/ |
/* Program reads a string from the serial port at 9600 bps 8N1 format */ |
/* Baudrate - 9600 */ |
/* Stop bits -1 */ |
/* No Parity */ |
/*----------------------------------------------------------------------------------------------------*/ |
/* Compiler/IDE : gcc 4.6.3 */ |
/* Library : */ |
/* Commands : gcc -o serialport_read serialport_read.c */ |
/* OS : Linux(x86) (Linux Mint 13 Maya)(Linux Kernel 3.x.x) */ |
/* Programmer : Rahul.S */ |
/* Date : 21-December-2014 */ |
/**/ |
/**/ |
/* www.xanthium.in */ |
/* Copyright (C) 2014 Rahul.S */ |
/**/ |
/**/ |
/* Running the executable */ |
/* ---------------------------------------------------------------------------------------------------*/ |
/* 1) Compile the serialport_read.c file using gcc on the terminal (without quotes) */ |
/**/ |
/* ' gcc -o serialport_read serialport_read.c ' */ |
/**/ |
/* 2) Linux will not allow you to access the serial port from user space,you have to be root.So use */ |
/* 'sudo' command to execute the compiled binary as super user. */ |
/**/ |
/* 'sudo ./serialport_read' */ |
/**/ |
/**/ |
/**/ |
/* Sellecting the Serial port Number on Linux */ |
/* ---------------------------------------------------------------------------------------------------*/ |
/* /dev/ttyUSBx - when using USB to Serial Converter, where x can be 0,1,2..etc */ |
/* /dev/ttySx - for PC hardware based Serial ports, where x can be 0,1,2..etc */ |
/**/ |
/*-------------------------------------------------------------*/ |
/* termios structure - /usr/include/asm-generic/termbits.h */ |
/* use 'man termios' to get more info about termios structure */ |
/*-------------------------------------------------------------*/ |
#include<stdio.h> |
#include<fcntl.h>/* File Control Definitions */ |
#include<termios.h>/* POSIX Terminal Control Definitions */ |
#include<unistd.h>/* UNIX Standard Definitions */ |
#include<errno.h>/* ERROR Number Definitions */ |
voidmain(void) |
{ |
int fd;/*File Descriptor*/ |
printf('n +----------------------------------+'); |
printf('n | Serial Port Read |'); |
printf('n +----------------------------------+'); |
/*------------------------------- Opening the Serial Port -------------------------------*/ |
/* Change /dev/ttyUSB0 to the one corresponding to your system */ |
fd = open('/dev/ttyUSB0',O_RDWR | O_NOCTTY); /* ttyUSB0 is the FT232 based USB2SERIAL Converter */ |
/* O_RDWR - Read/Write access to serial port */ |
/* O_NOCTTY - No terminal will control the process */ |
/* Open in blocking mode,read will wait */ |
if(fd -1) /* Error Checking */ |
printf('n Error! in Opening ttyUSB0 '); |
else |
printf('n ttyUSB0 Opened Successfully '); |
/*---------- Setting the Attributes of the serial port using termios structure --------- */ |
struct termios SerialPortSettings; /* Create the structure */ |
tcgetattr(fd, &SerialPortSettings); /* Get the current attributes of the Serial port */ |
/* Setting the Baud rate */ |
cfsetispeed(&SerialPortSettings,B9600); /* Set Read Speed as 9600 */ |
cfsetospeed(&SerialPortSettings,B9600); /* Set Write Speed as 9600 */ |
/* 8N1 Mode */ |
SerialPortSettings.c_cflag &= ~PARENB; /* Disables the Parity Enable bit(PARENB),So No Parity */ |
SerialPortSettings.c_cflag &= ~CSTOPB; /* CSTOPB = 2 Stop bits,here it is cleared so 1 Stop bit */ |
SerialPortSettings.c_cflag &= ~CSIZE; /* Clears the mask for setting the data size */ |
SerialPortSettings.c_cflag |= CS8; /* Set the data bits = 8 */ |
SerialPortSettings.c_cflag &= ~CRTSCTS; /* No Hardware flow Control */ |
SerialPortSettings.c_cflag |= CREAD | CLOCAL; /* Enable receiver,Ignore Modem Control lines */ |
SerialPortSettings.c_iflag &= ~(IXON | IXOFF | IXANY); /* Disable XON/XOFF flow control both i/p and o/p */ |
SerialPortSettings.c_iflag &= ~(ICANON | ECHO | ECHOE | ISIG); /* Non Cannonical mode */ |
SerialPortSettings.c_oflag &= ~OPOST;/*No Output Processing*/ |
/* Setting Time outs */ |
SerialPortSettings.c_cc[VMIN] = 10; /* Read at least 10 characters */ |
SerialPortSettings.c_cc[VTIME] = 0; /* Wait indefinetly */ |
if((tcsetattr(fd,TCSANOW,&SerialPortSettings)) != 0) /* Set the attributes to the termios structure*/ |
printf('n ERROR ! in Setting attributes'); |
else |
printf('n BaudRate = 9600 n StopBits = 1 n Parity = none'); |
/*------------------------------- Read data from serial port -----------------------------*/ |
tcflush(fd, TCIFLUSH); /* Discards old data in the rx buffer */ |
char read_buffer[32]; /* Buffer to store the data received */ |
int bytes_read = 0; /* Number of bytes read by the read() system call */ |
int i = 0; |
bytes_read = read(fd,&read_buffer,32); /* Read the data */ |
printf('nn Bytes Rxed -%d', bytes_read); /* Print the number of bytes read */ |
printf('nn'); |
for(i=0;i<bytes_read;i++) /*printing only the received characters*/ |
printf('%c',read_buffer[i]); |
printf('n +----------------------------------+nnn'); |
close(fd); /* Close the serial port */ |
} |
Copy lines Copy permalink
Definition
Examples
The following code example demonstrates the use of the SerialPort class to allow two users to chat from two separate computers connected by a null modem cable. In this example, the users are prompted for the port settings and a username before chatting. Both computers must be executing the program to achieve full functionality of this example.
Remarks
Use this class to control a serial port file resource. This class provides synchronous and event-driven I/O, access to pin and break states, and access to serial driver properties. Additionally, the functionality of this class can be wrapped in an internal Stream object, accessible through the BaseStream property, and passed to classes that wrap or use streams.
The SerialPort class supports the following encodings: ASCIIEncoding, UTF8Encoding, UnicodeEncoding, UTF32Encoding, and any encoding defined in mscorlib.dll where the code page is less than 50000 or the code page is 54936. You can use alternate encodings, but you must use the ReadByte or Write method and perform the encoding yourself.
You use the GetPortNames method to retrieve the valid ports for the current computer.
If a SerialPort object becomes blocked during a read operation, do not abort the thread. Instead, either close the base stream or dispose of the SerialPort object.
Constructors
SerialPort()SerialPort()SerialPort()SerialPort() | Initializes a new instance of the SerialPort class. |
SerialPort(IContainer)SerialPort(IContainer)SerialPort(IContainer)SerialPort(IContainer) | Initializes a new instance of the SerialPort class using the specified IContainer object. |
SerialPort(String)SerialPort(String)SerialPort(String)SerialPort(String) | Initializes a new instance of the SerialPort class using the specified port name. |
SerialPort(String, Int32)SerialPort(String, Int32)SerialPort(String, Int32)SerialPort(String, Int32) | Initializes a new instance of the SerialPort class using the specified port name and baud rate. |
SerialPort(String, Int32, Parity)SerialPort(String, Int32, Parity)SerialPort(String, Int32, Parity)SerialPort(String, Int32, Parity) | Initializes a new instance of the SerialPort class using the specified port name, baud rate, and parity bit. |
SerialPort(String, Int32, Parity, Int32)SerialPort(String, Int32, Parity, Int32)SerialPort(String, Int32, Parity, Int32)SerialPort(String, Int32, Parity, Int32) | Initializes a new instance of the SerialPort class using the specified port name, baud rate, parity bit, and data bits. |
SerialPort(String, Int32, Parity, Int32, StopBits)SerialPort(String, Int32, Parity, Int32, StopBits)SerialPort(String, Int32, Parity, Int32, StopBits)SerialPort(String, Int32, Parity, Int32, StopBits) | Initializes a new instance of the SerialPort class using the specified port name, baud rate, parity bit, data bits, and stop bit. |
Fields
InfiniteTimeoutInfiniteTimeoutInfiniteTimeoutInfiniteTimeout | Indicates that no time-out should occur. |
Properties
C Sharp Serial Port Example
BaseStreamBaseStreamBaseStreamBaseStream | Gets the underlying Stream object for a SerialPort object. |
BaudRateBaudRateBaudRateBaudRate | Gets or sets the serial baud rate. |
BreakStateBreakStateBreakStateBreakState | Gets or sets the break signal state. The office uk episode 1 season 1. |
BytesToReadBytesToReadBytesToReadBytesToRead | Gets the number of bytes of data in the receive buffer. |
BytesToWriteBytesToWriteBytesToWriteBytesToWrite | Gets the number of bytes of data in the send buffer. |
CanRaiseEventsCanRaiseEventsCanRaiseEventsCanRaiseEvents | Gets a value indicating whether the component can raise an event. (Inherited from Component) |
CDHoldingCDHoldingCDHoldingCDHolding | Gets the state of the Carrier Detect line for the port. |
ContainerContainerContainerContainer | Gets the IContainer that contains the Component. (Inherited from Component) |
CtsHoldingCtsHoldingCtsHoldingCtsHolding | Gets the state of the Clear-to-Send line. |
DataBitsDataBitsDataBitsDataBits | Gets or sets the standard length of data bits per byte. |
DesignModeDesignModeDesignModeDesignMode | Gets a value that indicates whether the Component is currently in design mode. (Inherited from Component) |
DiscardNullDiscardNullDiscardNullDiscardNull | Gets or sets a value indicating whether null bytes are ignored when transmitted between the port and the receive buffer. |
DsrHoldingDsrHoldingDsrHoldingDsrHolding | Gets the state of the Data Set Ready (DSR) signal. |
DtrEnableDtrEnableDtrEnableDtrEnable | Gets or sets a value that enables the Data Terminal Ready (DTR) signal during serial communication. |
EncodingEncodingEncodingEncoding | Gets or sets the byte encoding for pre- and post-transmission conversion of text. |
EventsEventsEventsEvents | Gets the list of event handlers that are attached to this Component. (Inherited from Component) |
HandshakeHandshakeHandshakeHandshake | Gets or sets the handshaking protocol for serial port transmission of data using a value from Handshake. |
IsOpenIsOpenIsOpenIsOpen | Gets a value indicating the open or closed status of the SerialPort object. |
NewLineNewLineNewLineNewLine | Gets or sets the value used to interpret the end of a call to the ReadLine() and WriteLine(String) methods. |
ParityParityParityParity | Gets or sets the parity-checking protocol. |
ParityReplaceParityReplaceParityReplaceParityReplace | Gets or sets the byte that replaces invalid bytes in a data stream when a parity error occurs. |
PortNamePortNamePortNamePortName | Gets or sets the port for communications, including but not limited to all available COM ports. |
ReadBufferSizeReadBufferSizeReadBufferSizeReadBufferSize | Gets or sets the size of the SerialPort input buffer. |
ReadTimeoutReadTimeoutReadTimeoutReadTimeout | Gets or sets the number of milliseconds before a time-out occurs when a read operation does not finish. |
ReceivedBytesThresholdReceivedBytesThresholdReceivedBytesThresholdReceivedBytesThreshold | Gets or sets the number of bytes in the internal input buffer before a DataReceived event occurs. |
RtsEnableRtsEnableRtsEnableRtsEnable | Gets or sets a value indicating whether the Request to Send (RTS) signal is enabled during serial communication. |
SiteSiteSiteSite | Gets or sets the ISite of the Component. (Inherited from Component) |
StopBitsStopBitsStopBitsStopBits | Gets or sets the standard number of stopbits per byte. |
WriteBufferSizeWriteBufferSizeWriteBufferSizeWriteBufferSize | Gets or sets the size of the serial port output buffer. |
WriteTimeoutWriteTimeoutWriteTimeoutWriteTimeout | Gets or sets the number of milliseconds before a time-out occurs when a write operation does not finish. Kingston ssdnow cloning software. SATA to AHCI (you said it ways already?)3. Apply overprovisioning to the SSD, and update its firmware7. Remove HDD for now5. Boot and test (may need to change the Boot Order in the motherboard BIOS)6. |
Methods
Close()Close()Close()Close() | Closes the port connection, sets the IsOpen property to |
CreateObjRef(Type)CreateObjRef(Type)CreateObjRef(Type)CreateObjRef(Type) | Creates an object that contains all the relevant information required to generate a proxy used to communicate with a remote object. (Inherited from MarshalByRefObject) |
DiscardInBuffer()DiscardInBuffer()DiscardInBuffer()DiscardInBuffer() | Discards data from the serial driver's receive buffer. |
DiscardOutBuffer()DiscardOutBuffer()DiscardOutBuffer()DiscardOutBuffer() | Discards data from the serial driver's transmit buffer. |
Dispose()Dispose()Dispose()Dispose() | Releases all resources used by the Component. (Inherited from Component) |
Dispose(Boolean)Dispose(Boolean)Dispose(Boolean)Dispose(Boolean) | Releases the unmanaged resources used by the SerialPort and optionally releases the managed resources. |
Equals(Object)Equals(Object)Equals(Object)Equals(Object) | Determines whether the specified object is equal to the current object. (Inherited from Object) |
GetHashCode()GetHashCode()GetHashCode()GetHashCode() | Serves as the default hash function. (Inherited from Object) |
GetLifetimeService()GetLifetimeService()GetLifetimeService()GetLifetimeService() | Retrieves the current lifetime service object that controls the lifetime policy for this instance. (Inherited from MarshalByRefObject) |
GetPortNames()GetPortNames()GetPortNames()GetPortNames() | Gets an array of serial port names for the current computer. |
GetService(Type)GetService(Type)GetService(Type)GetService(Type) | Returns an object that represents a service provided by the Component or by its Container. (Inherited from Component) |
GetType()GetType()GetType()GetType() | Gets the Type of the current instance. (Inherited from Object) |
InitializeLifetimeService()InitializeLifetimeService()InitializeLifetimeService()InitializeLifetimeService() | Obtains a lifetime service object to control the lifetime policy for this instance. (Inherited from MarshalByRefObject) |
MemberwiseClone()MemberwiseClone()MemberwiseClone()MemberwiseClone() | Creates a shallow copy of the current Object. (Inherited from Object) |
MemberwiseClone(Boolean)MemberwiseClone(Boolean)MemberwiseClone(Boolean)MemberwiseClone(Boolean) | Creates a shallow copy of the current MarshalByRefObject object. (Inherited from MarshalByRefObject) |
Open()Open()Open()Open() | Opens a new serial port connection. |
Read(Byte[], Int32, Int32)Read(Byte[], Int32, Int32)Read(Byte[], Int32, Int32)Read(Byte[], Int32, Int32) | Reads a number of bytes from the SerialPort input buffer and writes those bytes into a byte array at the specified offset. |
Read(Char[], Int32, Int32)Read(Char[], Int32, Int32)Read(Char[], Int32, Int32)Read(Char[], Int32, Int32) | Reads a number of characters from the SerialPort input buffer and writes them into an array of characters at a given offset. |
ReadByte()ReadByte()ReadByte()ReadByte() | Synchronously reads one byte from the SerialPort input buffer. |
ReadChar()ReadChar()ReadChar()ReadChar() | Synchronously reads one character from the SerialPort input buffer. |
ReadExisting()ReadExisting()ReadExisting()ReadExisting() | Reads all immediately available bytes, based on the encoding, in both the stream and the input buffer of the SerialPort object. |
ReadLine()ReadLine()ReadLine()ReadLine() | Reads up to the NewLine value in the input buffer. |
ReadTo(String)ReadTo(String)ReadTo(String)ReadTo(String) | Reads a string up to the specified |
ToString()ToString()ToString()ToString() | Returns a String containing the name of the Component, if any. This method should not be overridden. (Inherited from Component) |
Write(Byte[], Int32, Int32)Write(Byte[], Int32, Int32)Write(Byte[], Int32, Int32)Write(Byte[], Int32, Int32) | Writes a specified number of bytes to the serial port using data from a buffer. |
Write(Char[], Int32, Int32)Write(Char[], Int32, Int32)Write(Char[], Int32, Int32)Write(Char[], Int32, Int32) | Writes a specified number of characters to the serial port using data from a buffer. |
Write(String)Write(String)Write(String)Write(String) | Writes the specified string to the serial port. |
WriteLine(String)WriteLine(String)WriteLine(String)WriteLine(String) | Writes the specified string and the NewLine value to the output buffer. |
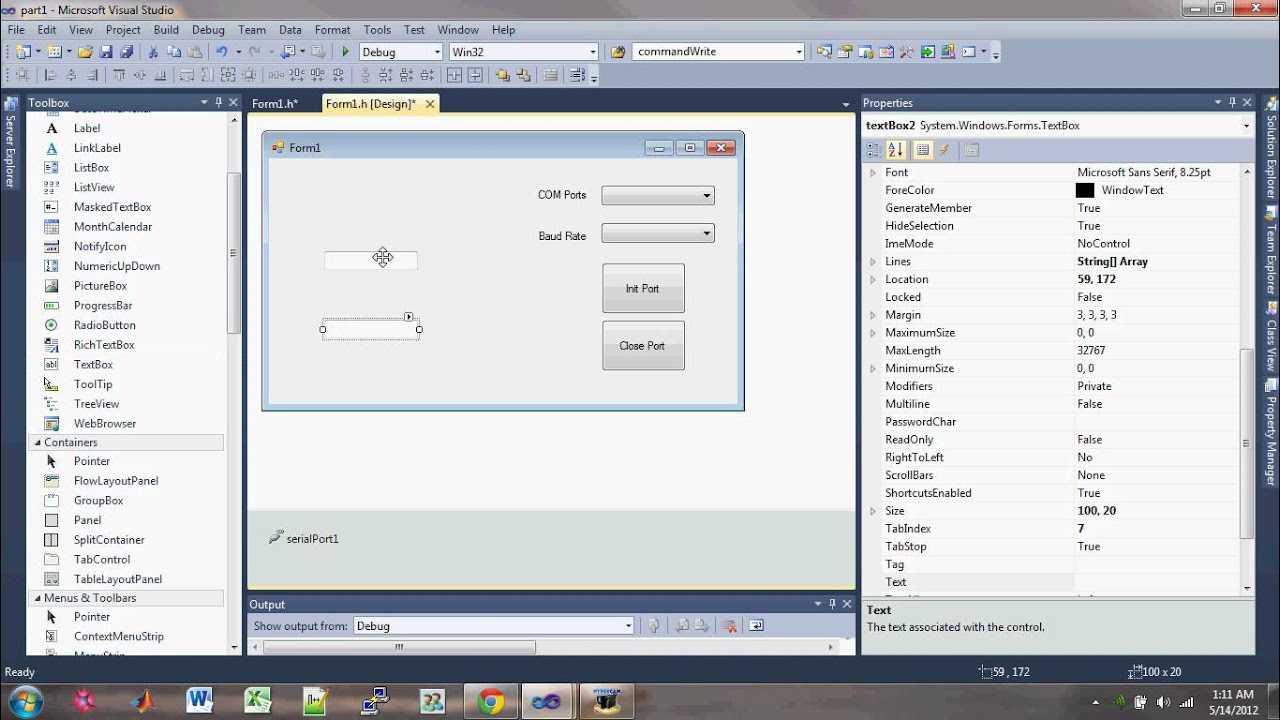
Events
DataReceivedDataReceivedDataReceivedDataReceived | Indicates that data has been received through a port represented by the SerialPort object. |
DisposedDisposedDisposedDisposed | Occurs when the component is disposed by a call to the Dispose() method. (Inherited from Component) |
ErrorReceivedErrorReceivedErrorReceivedErrorReceived | Indicates that an error has occurred with a port represented by a SerialPort object. |
PinChangedPinChangedPinChangedPinChanged | Indicates that a non-data signal event has occurred on the port represented by the SerialPort object. |
Security
C# Serial Port Example Code
SecurityPermission
for the ability to call unmanaged code. Associated enumeration: UnmanagedCode